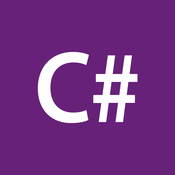
Swap value between two variables in C#
One of the first challenges when we start to learn programming, after Hello world of course :), is swapping a variable and Swap value between two variables in C#.
Like almost every programming problem, this one as well has several solutions. Some more effective than others.
Although one of the solutions for this one can be very easy, the other two that will be presented here, require little more thinking outside of the box.
Lets start with the first one!
First and the easiest method is with using third variable. Inside this variable we temporary keep the value of one of the variables that need to be swapped.
public static void Swap_with_third_variable(ref int a, ref int b)
{
// For this example we will consider that a=5, and b=3
int temp = a; // we create a temporary variable in memory for keeping the value of a
a = b; // here a becomes 3
b = temp; // b gets the value of temp and now b=5
// at the end we have a=3, b=5
}
The second solution involves little mathematics 🙂 It is very simple though, but I bet you didn’t think of it. It basically uses addition and subtraction.
public static void Swap_with_no_third_variable(ref int a, ref int b)
{
// for example we will consider that a=3, and b=5
a = a + b; // the value of a here will become 3+5 = 8
b = a - b; // the value of b will be 8-5=3;
a = a - b; // the value of a will be 8-3(note how new value of b is 3 already from the previous step)=5
// at the and we have a=5, b=3
}
Of course this will work with not only addition and subtraction, but with multiplication and division as well. Just be careful, if some variable has a value of = 0, forget about it 🙂 You know what is the result of X * 0, or X / 0 :). So use this only for testing and demonstration purposes.
The third solution is called bitwise. Although I will post the code for it, I will not say a word for it. Instead I will try to wake up your curiosity and ask you to try to explain how it works.
public static void Swap_bitwise(ref int a, ref int b)
{
a ^= b;
b ^= a;
a ^= b;
}
Complete project that can be tested with Visual Studio 2010. The project files can be downloaded as a zip file from here.
Tip for improving Your problem solving capabilities. In my career as a developer I have been faced with problems with different complexity levels. Some much harder than others, but I have to solve all of them :). My expertise is mainly oriented in C#.Net, but the secret and my tip for You is: try to solve the same problems in few different languages, or at least find a solution on the problems in several different languages and inspect the code in detail. Different languages have different capabilities, some of them are more powerful than others. Some of them have a different approach than others. This will help You to improve Your logic and You will eventually start thinking outside of the box when faced with future problems.